VueX State Management with Axios POST API Calls Made Easy
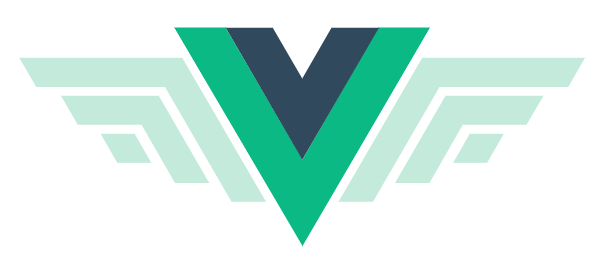
VueX is the powerful extension of Vue which helps us harness the power of caching. And Axios is wrapper class for javascript fetch method, mostly preferred by developers and suggested by Vue. POST requests in VueCLI take a new form via Axios and can cause the development process to go slow.
Saving the data in VueX on each step of the data input process and calling the API may be a challenging task for beginners. Saving the data in VueX is a good practice as it can be used later anytime until the user does not reload the website. This is extremely beneficial in web-apps made via Vue as almost all navigation is done via the router links the app.
Setting up store.js for Vue Management
The following adds the Vuex storage for the class. Make sure to import the same first.
export default new Vuex.Store({})//adding data in store
state: {
userDetails: {
email: '',
password: '',
}
},// this works similar to key value data storage but not limited to only text.//mutations is the place where all API calls and data manipulation ins donemutations: {
LOGIN_USER: (state) => {
}
},//actions for a way to provide acces to mutations via Vue Components
actions: {
loginUser(context){
//commit message tells which mutation to execute
context.commit("LOGIN_USER")
}
},//make sure all data in the store is sent via getters and not directly
getters: {
getUserDetails: state => {
return state.userDetails.email
}
}
After adding all the data in Vuex.store, we are now ready to reference them in VueCli components and make API Calls.
Saving the data via Components
In the script part of the components, declare the variables in the data() part and you can update them via v-model attached with inputs. The data saving is done via functions declared in methods segment. Eg:
methods: {
saveEmail: function (email) {
this.$store.state.userDetails.email = email
}
},
The following example saves the email to the store state we declared in the first step. Make sure to call this function on any event as required. Eg.
@click="saveEmail(e_mail)"
The following function is called on clicking the element, mostly a submit button. Here the e_mail parameter passed is declared in the data segment of the script component.
Making the API Call with Axios
This is the tricky part because of the extra headers we have to send to the API call. In the store.js add the following to a mutation declared in the 3rd code above.
//declare the body form
let loginFormData = new FormData();
//add the parameters
loginFormData.set('email', state.userDetails.email);
loginFormData.set('password', state.userDetails.password);
const config = {
headers: {
'Content-Type': `multipart/form-data; boundary=${loginFormData._boundary}`
}
};//important step
Axios.post('url', loginFormData, config)
.then(data => {
console.log(data.data);
//handle the data
})
.catch(error => {
console.log(error.response)
})
The set method may be replaced with append to send any files or images. Also, some users if facing issue with set can replace it with .append, with same function call definition.
The content type header is an important step for the POST request. Though it is not explicitly explained anywhere why the following works, but for API calls in VueCli make sure to add the following header and boundary with the same syntax to actually sending the data. Otherwise, Axios may send empty data to the server returning errors.
headers: {
‘Content-Type’: `multipart/form-data; boundary=${loginFormData._boundary}`
}
Calling the API function in the component
Remember we created actions for calling mutations. Now we call these actions via the components. Add the following in the methods segment of the CLI script
loginUser: function (email, password) {
this.$store.dispatch('loginUser')
.then((result) => {
//handle the returened data
})
},
Remember the following method is called in the same way we called via saving the data in the store.
It covers most of the issues happening with the vuex store and Axios Post requests.
Note:
If you’re getting CORS error in the console, it means the browser is not able to hit the URL because the web-app is on your local system. Download the following chrome extension:
and add the format of the API URL to be accessed and turn it on before sending the request.